[Android studio] SharedPreferences를 이용한 데이터 저장하기 로컬 데이터 베이스
개발을 진행하다 보면 애플리케이션이 꺼졌다 켜져도 데이터가 유지되는 것을 원할 때가 있다.
DB를 사용하기에는 작은 규모의 데이터일 때 SharedPreferences를 이용하여 간단하게 사용할 수 있다.
우선 PreferenceManager.class를 생성하자
public class PreferenceManager {
public static final String PREFERENCES_NAME = "rebuild_preference";
private static final String DEFAULT_VALUE_STRING = "";
private static final boolean DEFAULT_VALUE_BOOLEAN = false;
private static final int DEFAULT_VALUE_INT = -1;
private static final long DEFAULT_VALUE_LONG = -1L;
private static final float DEFAULT_VALUE_FLOAT = -1F;
private static SharedPreferences getPreferences(Context context) {
return context.getSharedPreferences(PREFERENCES_NAME, Context.MODE_PRIVATE);
}
/**
* String 값 저장
* @param context
* @param key
* @param value
*/
public static void setString(Context context, String key, String value) {
SharedPreferences prefs = getPreferences(context);
SharedPreferences.Editor editor = prefs.edit();
editor.putString(key, value);
editor.commit();
}
/**
* boolean 값 저장
* @param context
* @param key
* @param value
*/
public static void setBoolean(Context context, String key, boolean value) {
SharedPreferences prefs = getPreferences(context);
SharedPreferences.Editor editor = prefs.edit();
editor.putBoolean(key, value);
editor.commit();
}
/**
* int 값 저장
* @param context
* @param key
* @param value
*/
public static void setInt(Context context, String key, int value) {
SharedPreferences prefs = getPreferences(context);
SharedPreferences.Editor editor = prefs.edit();
editor.putInt(key, value);
editor.commit();
}
/**
* long 값 저장
* @param context
* @param key
* @param value
*/
public static void setLong(Context context, String key, long value) {
SharedPreferences prefs = getPreferences(context);
SharedPreferences.Editor editor = prefs.edit();
editor.putLong(key, value);
editor.commit();
}
/**
* float 값 저장
* @param context
* @param key
* @param value
*/
public static void setFloat(Context context, String key, float value) {
SharedPreferences prefs = getPreferences(context);
SharedPreferences.Editor editor = prefs.edit();
editor.putFloat(key, value);
editor.commit();
}
/**
* String 값 로드
* @param context
* @param key
* @return
*/
public static String getString(Context context, String key) {
SharedPreferences prefs = getPreferences(context);
String value = prefs.getString(key, DEFAULT_VALUE_STRING);
return value;
}
/**
* boolean 값 로드
* @param context
* @param key
* @return
*/
public static boolean getBoolean(Context context, String key) {
SharedPreferences prefs = getPreferences(context);
boolean value = prefs.getBoolean(key, DEFAULT_VALUE_BOOLEAN);
return value;
}
/**
* int 값 로드
* @param context
* @param key
* @return
*/
public static int getInt(Context context, String key) {
SharedPreferences prefs = getPreferences(context);
int value = prefs.getInt(key, DEFAULT_VALUE_INT);
return value;
}
/**
* long 값 로드
* @param context
* @param key
* @return
*/
public static long getLong(Context context, String key) {
SharedPreferences prefs = getPreferences(context);
long value = prefs.getLong(key, DEFAULT_VALUE_LONG);
return value;
}
/**
* float 값 로드
* @param context
* @param key
* @return
*/
public static float getFloat(Context context, String key) {
SharedPreferences prefs = getPreferences(context);
float value = prefs.getFloat(key, DEFAULT_VALUE_FLOAT);
return value;
}
/**
* 키 값 삭제
* @param context
* @param key
*/
public static void removeKey(Context context, String key) {
SharedPreferences prefs = getPreferences(context);
SharedPreferences.Editor edit = prefs.edit();
edit.remove(key);
edit.commit();
}
/**
* 모든 저장 데이터 삭제
* @param context
*/
public static void clear(Context context) {
SharedPreferences prefs = getPreferences(context);
SharedPreferences.Editor edit = prefs.edit();
edit.clear();
edit.commit();
}
}
이제 본격적으로 데이터를 저장할 것인데 메인 액티비티에서 아래 코드를 입력해보자
PreferenceManager.setString(getApplicationContext(), "키값", "저장할 값");
필자는 우선 String형을 저장하는 예제를 작성했다.
키값과 저장할 값에 대해 이해하기 쉽게 예를 들어보겠다.
데이터를 사람이라고 생각해보자 그 사람의 이름은 김 OO이다
저기 멀리서 김 OO 씨 뛰어오세요!라고 하면 김 OO 씨의 몸이 뛰어오는 것이다.
여기서 김 OO 씨라는 이름은 키값 김 OO 씨의 뛰어오는 몸은 저장할 값이 되는 것이다.
저장한 데이터를 가져올 때는 김 OO 씨 이름만 알면 그 값을 가져올 수 있는 것이다.
아래와 같이 다시 작성해보자
//데이터 저장
String str = "나이 : 36세 성별 : 남자";
PreferenceManager.setString(getApplicationContext(), "kim",str);
//데이터 호출
String kim = PreferenceManager.getString(getApplicationContext(), "kim");
Toast.makeText(getApplicationContext(), kim, Toast.LENGTH_SHORT).show();
str 변수의 값을 "kim"이라는 키값으로 저장한 후 "kim"이라는 키값에 해당하는 값을 다시 호출했다.
다른 데이터 형식의 값도 저장할 수 있다.
주의할 점으로 SharedPreferences를 사용하면 스마트폰 로컬에 데이터가 남는다.
이 데이터를 열어볼 수 있으므로 보안에 취약하다.
유저의 개인정보와 같은 보안상 중요한 데이터는 SharedPreferences를 사용하여 저장하지 않도록 조심해야 한다.
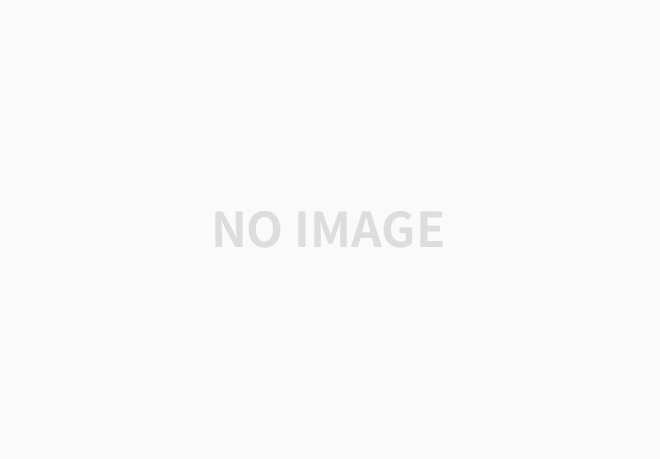
'APP > AndroidStudio' 카테고리의 다른 글
[Android studio] 버튼클릭 지점부터 물결효과 주기 Button ripple 안드로이드 스튜디오 (0) | 2020.07.15 |
---|---|
[Android Studio] RecyclerView 리사이클러뷰 사용하기 리스트뷰 (0) | 2020.07.12 |
[Android Studio] 버튼 누르는 효과 만들기 클릭 이벤트 효과 Button Press 안드로이드 스튜디오 (0) | 2020.07.06 |
[Android Studio] 버튼 둥글게 만들기 안드로이드 스튜디오 Button Round (0) | 2020.07.03 |
[Android Studio] 간단하게 Fragment에서 getContentResolver() 사용하기 getApplicationContext() (0) | 2020.06.30 |